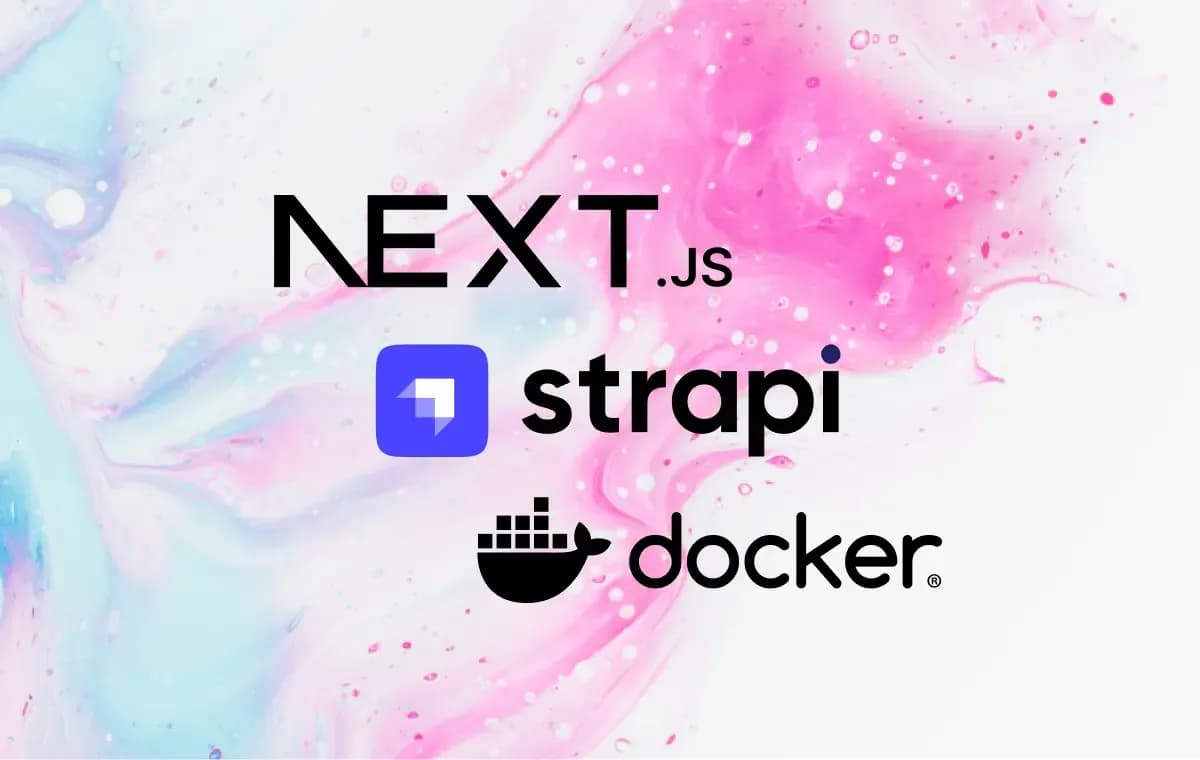
How to Build a Dynamic Website with Strapi, Next.js, Postgres and Docker
I built this website using NextJS (with TypeScript and TailwindCSS) for the front-end, a self-hosted Strapi CMS with Postgres for content management and Docker for containerization and deployment on an Ubuntu VPS.
Introduction
In this guide, we'll set up a dynamic website using modern web development tools: Strapi, Next.js, and Docker. These tools offer a powerful blend of flexibility, efficiency, and scalability, empowering you to craft a professional-grade website that not only showcases your work but also streamlines content publishing and updating your deployments.
##Key Concepts
If you are unfamiliar with the concept of a headless CMS, the diagram below helps to quickly explain the concept. Gone are the days of monolithic content management applications. Sayonara! What makes a headless CMS cool is that it is decoupled from front-end and consuming our content is done via API. For more details, check out the Complete Guide to Headless CMS. If you're interested in the other options available, you can review the available options
- Part One: Back-end with Strapi, Postgres & Docker
- Part Two: Front-end with Next.js, TypeScript and TailwindCSS
Additionally, I've opted to integrate TypeScript into our stack. TypeScript enhances JavaScript with static typing, ensuring early error detection and fostering code maintainability and scalability. While it may pose some initial challenges, the long-term benefits are undeniable. Trust me, you'll appreciate it down the road.
The Technology Stack
Next.js: Next.js, a React framework, seamlessly blends server-side and client-side rendering, offering an intuitive environment for crafting dynamic web applications.
Strapi: Strapi serves as our open-source headless CMS (Content Management System), providing a flexible backend for content management.
Postgres: PostgreSQL, renowned for its reliability and scalability, serves as our robust open-source relational database management system (RDBMS).
Docker: Docker, with its containerization technology, facilitates the packaging of applications and dependencies into lightweight containers, ensuring consistent deployment across diverse environments.
Node.js: Node.js, a powerful JavaScript runtime environment, empowers developers to build scalable, server-side applications.
Nginx: Nginx, a high-performance web server and reverse proxy server, excels in efficiently handling web traffic.
Setup
⚠️ Note: The following setup instructions are tailored for Mac users.
###Prerequisites
- Mac machine
I utilize a Virtual Private Server (VPS) running Ubuntu for deployment, along with a set of tools to streamline the production process. We'll delve into this setup later; for now, let's focus on configuring our local development environment.
I rely on Visual Studio Code (VS Code), supplemented with React and TypeScript plugins for efficient coding. Additionally, Strapi and Next.js come equipped with tools to streamline development. To expedite styling, I leverage Tailwind CSS. We'll detail this later in the setup.
To host your repositories for front-end (Next.js) and back-end (Strapi) development, you'll need a GitHub account, which we'll integrate for automatic deployment upon branch updates.
Local Development Environment
Let's kickstart our local environment setup by installing the necessary packages, starting with Strapi. Referencing the Strapi documentation, ensure your system meets the following prerequisites:
- Node.js (Active LTS or Maintenance LTS versions, currently v18 and v20)
- Preferred Node.js package manager: npm (v6 and above) or yarn
Installing Node Version Manager
I advocate for the use of Node Version Manager (nvm) to manage multiple Node installations seamlessly. On a Mac, you can install nvm via Homebrew—a convenient package management system for macOS and Linux.
To begin, install Homebrew by executing the following command in your terminal:
/bin/bash -c "$(curl -fsSL https://raw.githubusercontent.com/Homebrew/install/master/install.sh)"
Once Homebrew is installed, proceed to install nvm with these commands:
brew update
brew install nvm
You may need to configure your bash terminal by editing the ~/.bash_profile file. Detailed instructions can be found here.
With nvm set up, you're ready to install the required Node.js versions for Strapi.
Install Required Node Version
To install the required node version, run the following:
nvm install v18.17.1
After installation and in the root of your project, run the following command:
nvm use v18.17.1
We will come back to the node version and nvm and create the .nvmrc file to persist the versioning later.
Install Docker and Postgres
Open VS code and create a new project directory. The is for the Strapi backend. Strapi provides a customizable front-end for our content management which means you can set it up and create your object schema for your database directly in the browser. But hold up - what about the database? Well, before we install Strapi, we will begin with getting a Postgres docker container up and running locally as we want to mimic our production environment as closely as possible.
Install Docker Desktop for your Mac Navigate to the terminal, do a docker pull from the official Postgres repository with the following:
docker pull postgres
Now that we have the image in our local environment, we can spin up a container and specify some parameters. The following configuration will be used to create a containerised instance of Postgres:
docker run
--name strapiPostgresDB
-p 5455:5432
-e POSTGRES_USER=strapi
-e POSTGRES_PASSWORD=password
-e POSTGRES_DB=postgresDB
-d
postgres
After executing this command, navigate to your Docker Desktop and under the containers tab you will be able to see your newly created 'strapiPostgresDB' container running.
Installing Strapi CLI
Now that we've set up our environment, let's install the Strapi CLI and create our project.
Installing Strapi CLI
In your terminal from within your project directory from earlier, run the following command to install the Strapi CLI globally:
npm install -g strapi@latest
Once the installation is complete, you can verify that the Strapi CLI has been installed correctly by running:
strapi --version
You should see the version number of the installed Strapi CLI.
Creating the Strapi Project
From within the root directory, run the following command to create a new Strapi project:
strapi new my-project
Replace my-project
with the name you want to give your project.
During the installation of Strapi, you will be asked some questions on your desired setup. The first question you'll be asked is to choose your database client. Strapi can be used with a variety of databases but we'll be using - surprise, surprise - Postgres. This will closely mimic our setup on our production environment and ensure that your mock data persists during local development.
Below is what you will see in your terminal. Enter the details we previously used to set up the Docker Postgres container:
? Choose your default database client postgres
? Database name: postgresDB
? Host: 127.0.0.1
? Port: 5455
? Username: strapi
? Password: ********
? Enable SSL connection: No
Managing Node Versions with .nvm
To ensure consistency in our Node.js environment, we'll create a .nvmrc
file at the root of our Strapi project directory. This file specifies the Node.js version required for our project.
Create a .nvmrc
file in the root of your newly created Strapi project and add the desired Node.js version. As of the time of writing, the latest version for Strapi is v18.17.1:
v18.17.1
Save and close the file.
Setting Node Version as Default
To make sure that the specified Node.js version is the default one used in our project, run the following command in the terminal:
nvm use
This command will switch to the Node.js version specified in the .nvmrc
file.
##Conclusion
Now you're all set! You have successfully installed Docker, spun up a containerised instance of Postgres, configured the Node.js environment for your projects, installed the Strapi CLI, created a new Strapi project, and connected it to the Postgres container.